Previously
I created a stock screener that gives a list of tickers that meets the requirements.
The requirements were used to find a stock that broke out last highest close price in 20 days.
The requirement i set is:
- last close price is bigger than the highest close price in 20 days (excluding last day)
If you are interested in python code, check out: https://mike-tyson.tistory.com/144
UI
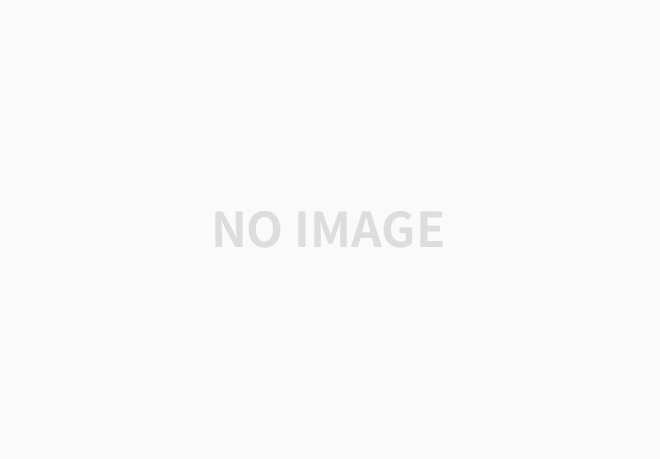
Procedure:
- python:
1. prepare result as such on UI
2. dump result onto a json file
- nextjs:
3. read json file
4. print data
1. prepare result as such on UI
data = yf.download(ticker, period="1mo")
highest_close_20_days = data['Close'][:-1].rolling(window=20).max()
last_close_price = data['Close'].iloc[-1].item()
highest_close_price = highest_close_20_days.iloc[-1].item()
if last_close_price > highest_close_price:
last_volume = data['Volume'].iloc[-1].item()
previous_volume = data['Volume'].iloc[-2].item()
volume_change_percentage = (last_volume - previous_volume) / previous_volume * 100
quotes.append(
{
"ticker": ticker,
"last_close_price": last_close_price,
"highest_close_price": highest_close_price,
"volume_change_percentage": volume_change_percentage
}
)
2. dump result onto a json file
data = quotes
with open('public/breaking_20_highest_close.json', 'w') as f:
json.dump(data, f)
Full python code:
import yfinance as yf
import pandas as pd
import json
nasdaq100_tickers = pd.read_html('https://en.wikipedia.org/wiki/Nasdaq-100#Components')[4]['Symbol']
#nasdaq100_tickers = nasdaq100_tickers[0:1]
quotes = []
for idx, ticker in enumerate(nasdaq100_tickers):
try:
remaining = len(nasdaq100_tickers) - idx - 1
print(f"checking quote {idx + 1}. remainding = {remaining}")
data = yf.download(ticker, period="1mo")
highest_close_20_days = data['Close'][:-1].rolling(window=20).max()
last_close_price = data['Close'].iloc[-1].item()
highest_close_price = highest_close_20_days.iloc[-1].item()
if last_close_price > highest_close_price:
last_volume = data['Volume'].iloc[-1].item()
previous_volume = data['Volume'].iloc[-2].item()
volume_change_percentage = (last_volume - previous_volume) / previous_volume * 100
quotes.append(
{
"ticker": ticker,
"last_close_price": last_close_price,
"highest_close_price": highest_close_price,
"volume_change_percentage": volume_change_percentage
}
)
except Exception as e:
print(f"Exception: {e}")
raise
print(f"total {len(quotes)} quotes found")
print(f"quotes: {quotes}")
data = quotes
with open('public/breaking_20_highest_close.json', 'w') as f:
json.dump(data, f)
3. read json file:
- warning: make sure you put the json inside /public/ directory
export async function getStaticProps() {
const res = await fetch('http://localhost:3000/breaking_20_highest_close.json');
const initialData = await res.json();
return {
props: {
initialData,
},
};
}
4. print data:
export default function Home({ initialData }) {
return (
<div>
<h1>Static Python Data</h1>
<pre>{JSON.stringify(initialData, null, 2)}</pre>
</div>
);
}
Full nextjs Code:
export default function Home({ initialData }) {
return (
<div>
<h1>Static Python Data</h1>
<pre>{JSON.stringify(initialData, null, 2)}</pre>
</div>
);
}
export async function getStaticProps() {
const res = await fetch('http://localhost:3000/breaking_20_highest_close.json');
const initialData = await res.json();
return {
props: {
initialData,
},
};
}
Result:
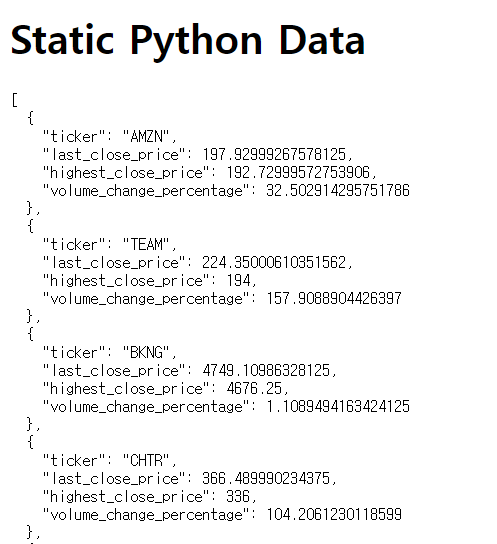